Steps to create an initial setup for a new TypeScript project

If you want to start creating a new React app or any JavaScript app using TypesScript, you can follow the below steps to create an initial setup for your application.
Let's get started!
Create a folder for your project and open a terminal inside your folder
Step 1: Let's create a package.json file inside our folder. For that, type the following command in your terminal
> npm init -y

Step 2: Now, let's create a tsconfig.json and also add 2 packages viz nodemon and concurrently ( We will require these packages later for running scripts )
> tsc --init && npm install nodemon concurrently
Now, you can see that inside your project folder, you can find node modules, package.json, tsconfig.json, and package-lock.json.
TypeScript project needs 2 main folders i.e src and build.
src is going to contain all our source code whereas the build will contain the complied TS code.
First, let's create them at the root level.
Create 2 folders, one for src and one for the build. We will take care of the src and build folder configuration in Step 4.
Step 3: Inside the src folder, let's create an index.tx file.
Add a simple console.log(`hello world`) inside index.js for now. Later you can replace it with some actual code.
Your project structure should look something like this

Step 4: Now, we are going to add some configuration inside our tsconfig.json file. tsconfig.json contains a tone of commented line. We are interested only in the following 2 commented lines for now.
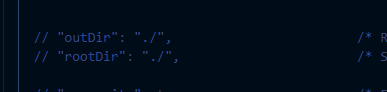
Uncomment them and replace them with

Step 5: Now, let's add some scripts inside our package.json
Replace the scripts in package.json with the following lines.
"scripts": {
"start:build": "tsc -w",
"start:run": "nodemon build/index.js",
"start": "concurrently npm:start:*",
}
start:run will listen and recompile anytime we add changes to our code and start will concurrently/together run all the scripts that starts with “start” as prefix (eg start:build, start:run )
Nows a time for a quick test. Head back to your terminal and write
> npm start
You might encounter an error at the first time saying

This is totally expected since nodemon will try to run index.js before typescript has successfully compiled it. Hit ctrl+c and again type
npm start inside the terminal.
This time, everything should run correctly and we can also get to see our console statement from our index.ts file.

Congratulation 🎉 We have now successfully build a setup for your new TypeScript project. Now go ahead and create some awesome applications!